How does a web app really work?
In this article we’ll dive into the details of how a web app works and the architecture required to make this happen. Let’s start by taking another look at the web app architecture diagram that we covered in the previous article.
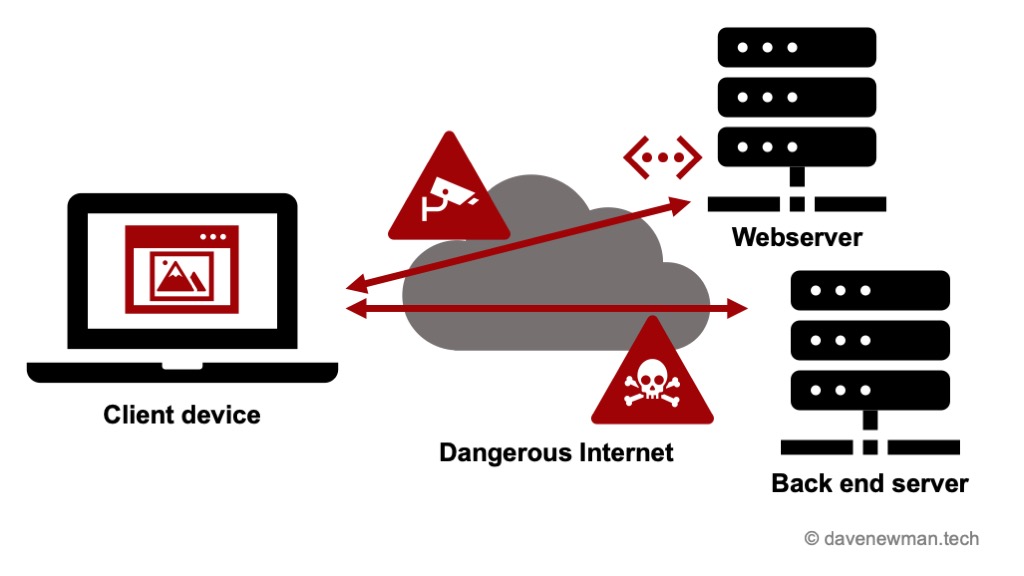
The client
The browser is the client. It downloads the code it needs to run for the web app from the webserver. This “code” is a mix of HTML pages, Javascript and CSS that are cached (saved) on the client machine and run in the browser. There are many frameworks that you can use to write this code. In this series I will be using React to create a Single Page Application (SPA).
One thing to be mindful of is that the code that you write for the client can be seen and ultimately analysed by anyone who has access to the client. For example some applications need to access data on another service (such as on Amazon Web Services) and that service requires a security key or password. That security key or password can not be embedded in your client code as anyone will be able to read your code and learn that secret information! You will need to use other methods to access those services from your clients. We’ll explore those methods later in this series.
Webserver
In the case of a web app the webserver only needs to serve the various files to the client. It doesn’t actually do any processing or generate any dynamic pages. It just needs to send the HTML, Javascript and CSS files to the client’s browser. The browser then runs the code from those files.
It is possible to use the same server as the back end as a webserver. But care will need to be taken as the webserver performs a different function and will have different needs from the back end.
The back end
The back end server provides many different services for the front end clients. It acts as the central repository for all data. It processes and transforms that data as necessary. It authenticates the users as they log in. Etc, etc.
Usually the back end functionality is split into 2 parts: the API server and the database server.
API server
Many back end servers are built following an Application Programming Interface (API) model. This is the server that the client interacts with. It provides the business logic for processing the data, etc. These services are provided as end points which can be thought of as a unique URL for each service. This model is called a RESTful API model. There are many articles that you can read to learn more about this model and I suggest you start with this article.
The API server itself doesn’t store your users data. For this we’ll need a database server.
Database server
There are many different databases that you can use to store your data. Common choices include MySQL and MongoDB. Depending on where you plan to host your back end your hosting provider may already have a database service included. For example, many managed hosting providers include MySQL in the hosting package. This makes it relatively easy to manage as you don’t need to install the database software on a barebones server.
Another option is to use a cloud database service like MongoDB Atlas. You can set up a database on their database servers and then configure your API server to connect to that database. This is also very easy to manage although the database size for the free trial is (obviously) limited. But it is a great way to get started.
Detailed architecture
Now let’s take a look at our detailed architecture diagram:
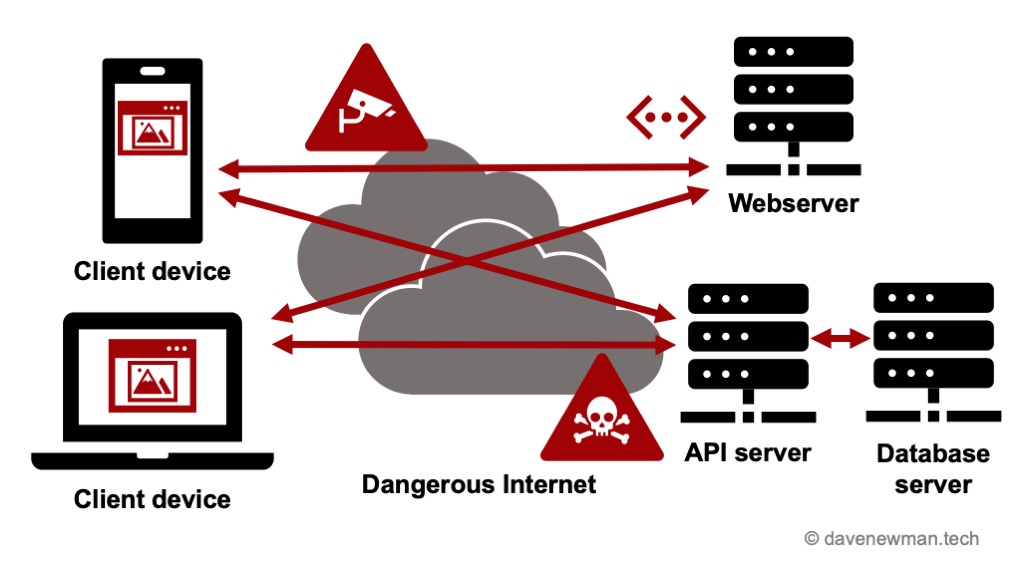
We can see that what was originally described as a “client-server” model is a lot more than just one client and one server. Now we have 3 different servers performing different services. But please don’t feel overwhelmed by this model! A lot of complexity on how these different services work together is managed by the frameworks that we will use to build the web app. React will manage the front end (the code running in the client’s browser), the webserver (building the HTML, Javascript and CSS files that the webserver needs to send to the client), and the interaction between the client and the API server. Node.js will also provide tools to manage the interaction between the client and the API server, and the tools to connect to the database server. Understanding this architecture model is a great start on your journey to being a full stack developer!
In the next article we will look at installing React and Node.js on a local machine for development and how that fits in with the web app architecture model.