Now that you have your Node API server running let’s get your React app hosted! This is simpler than you might think but first let’s look at what not to do…
Don’t set up a development web server for React
I have noticed that many, many people are asking how they should set up the development web server (localhost:3000) to run in their production environment. My first thought is Why? Why would you want to run a development server that is exposed to the world, vulnerable to any malicious hacker? Development servers are not secure and deliberately so. They are meant to provide you with lots of information when things go wrong so that you can debug your code. However, that information will give a malicious hacker clues on potential security holes that will put you and your users at risk. Just don’t do it!
Build a production version of your app
So what should you do instead? Create a production build of your React app. It’s not difficult – you just need to run the following instruction in your front end folder on your development machine:
npm run build
This will now build production-ready files for your server. All of the HTML, CSS, Javascript files will be generated as well as all other resources like images, etc will be put into appropriate subfolders.
How is this different from my development server?
The build process minifies your code making the Javascript files smaller. It also removes your comments, changes variable names, and so on, thus making it (slightly) harder for someone to reverse engineer your app.
Another thing it does is to put your code into Javascript files that can be cached in the client’s browser. This means the browser just needs to download it once and can keep on running those parts of your app if you don’t change the code. This will be a much faster and more efficient experience for your users.
Whereabouts on your server?
Then go to your Namecheap shared hosting server and decide where you will host your React app. You have a few choices:
- Host in the root directory of your server (e.g. www.example.com/ ). In this case just put all of the built files in the public_html folder
- Host in a subfolder (e.g. www.example.com/myapp/ ). You’ll need to create the “myapp” folder in the public_html folder and then copy across all the built files into that subfolder.
- Host in a subdomain (e.g. myapp.example.com/ ). Once you’ve created the subdomain using cPanel you’ll just need to copy across all of the built files into the appropriate folder (which will usually be off your /home/username folder, not the public_html). One thing to note is that your subdomain probably won’t be covered by the SSL certificate of your main domain unless you have a wildcard certificate. You’ll need to install another certificate for this subdomain if you want to use a secure https connection for your app (which you should).
I chose option 2 and hosted my React apps in subfolders. This is what the updated folder structure looks like (with the Node API back end running in another subfolder):
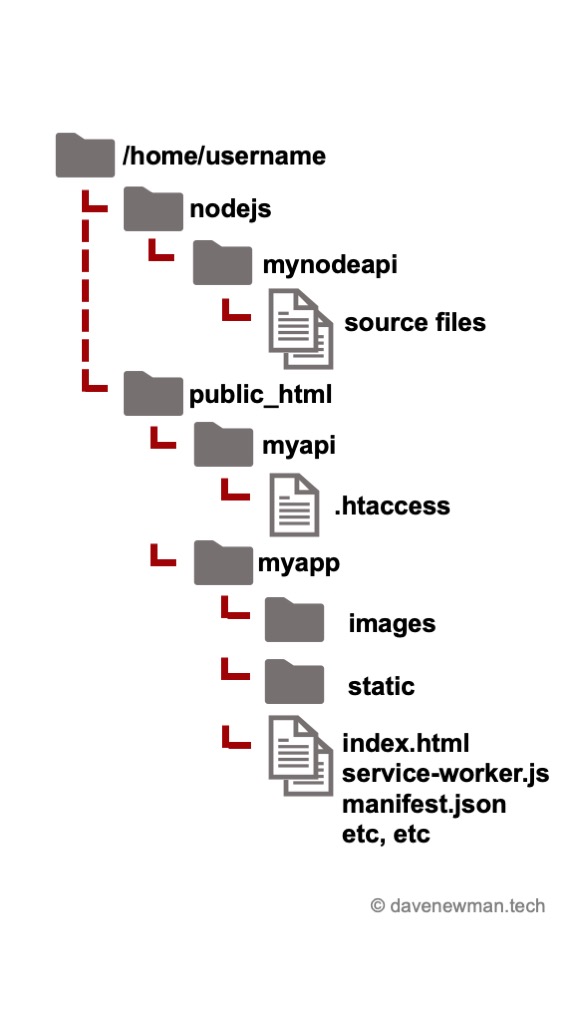
Ensuring a React Single Page Application works
The last step we need to do is to modify the .htaccess file in the same folder as the built files. We need to ensure that all of the requests will be answered by the index file especially when the user hits the reload button in their browser. The changes for a React app in a subfolder is:
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /
RewriteRule ^index\.html$ - [L]
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteCond %{REQUEST_FILENAME} !-l
RewriteRule ^.*$ /myapp/index.html [QSA,L]
</IfModule>
Just remove “/myapp” if you’re not running in a subfolder.
So what does it look like now?
Let’s take a look at the web app architecture diagram now:
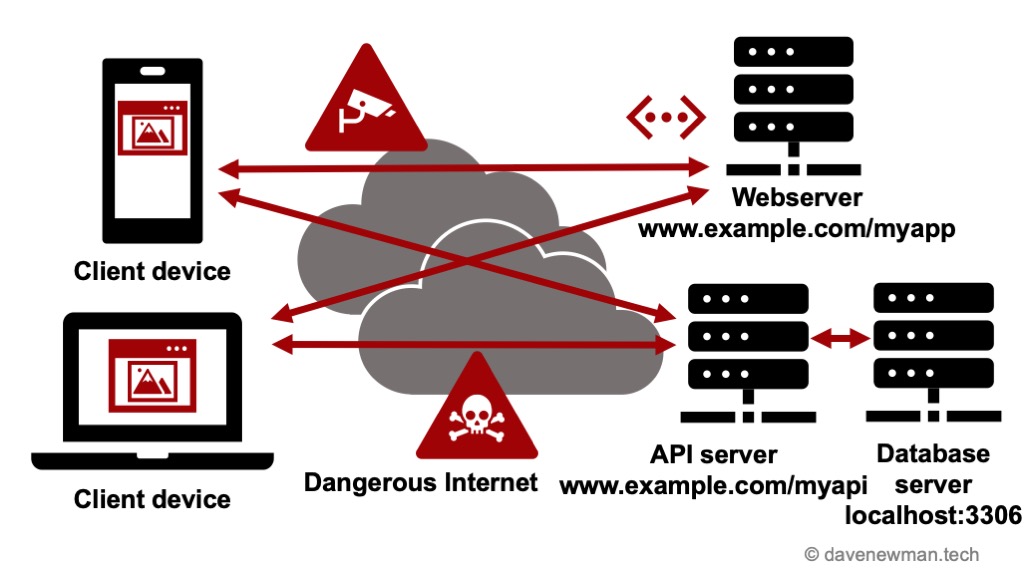
In this example we now have all of the servers running in Namecheap:
- The webserver serving the React app at example.com/myapp
- The Node API server at example.com/myapi
- And in this case the MySQL database also running on the same Namecheap shared hosting server and is accessible only by the localhost (the Node API server)
I hope this has helped you get started in hosting your Node and React app on a shared hosting platform like Namecheap! In the next article we will take a deeper dive at some of the settings for hosting in subfolders.
7 replies on “Hosting your React app on Namecheap”
Hi, thanks for the excellent article! Going through this right now with namecheap, and getting stuck (not really any useful help docs there!), but your article is helping provide a bit of light.
If I can ask… you have two folders, the myapi folder and the myapp folder. So myapi holds the path to the node app, and you would visit example.com/myapp in order to see this site? Where in your app do you point to the /myapi folder? It is in the frontend I am guessing? Is it possible to have both the myapp and myapi folder contents in the same main folder (public_html) or is there a functional reason for this (ie keeping the two .htaccess files separate)? Again, thanks for this article series, appreciate any insights you may have!
Thanks a lot for the feedback and questions Kat!
1. Yes, you would go to example.com/myapp in order to see the site.
2. In the React frontend code you may need to access data from your backend (and eventually from your database). The way you’d do that is to call a “fetch” command which would call your API backend. And effectively that’s a POST or GET HTTP call to your example.com/myapi.
The /myapi needs to be in a different folder from /myapp as the response will be handled by your Node.js app, not from the React app.
Thanks a lot for the question! It makes me think that I should write a more detailed post on how you call the API backend from the React frontend with some code snippets. Would that be helpful?
Hi Dave, on the previous tutorial you mentioned to put in RewriteEngine off, but in this one it’s now RewriteEngine On. Why is that and is this is the same .htaccess?
Thanks for the question Gerard!
We have 2 different .htaccess files in 2 different folders:
– One is for the Node.js backend. That should have the RewriteEngine off as our Node app will process the HTML request as it is.
– The other is for the React frontend. That needs to have the RewriteEngine on and effective redirects all of the HTML requests to index.html. That’s because we want to make sure that index.html will process the request which is the entry point for React’s javascript.
Hope that helps.
Do I have to run serve -s build after uploading the files to /myapp?
All I get is a blank page with the “React App” tab title.
Hello Anon,
Did you get any solution for this problem? I’m also facing the same problem. If you got any solution, could you help me about that?
I know this is an old comment but just in case anyone else runs into this: check the developer console, in my case I had a bunch of 404s. The index.html that was autogenerated by the sample react project was using paths relative to root. In my case prepending them with a “.” fixed the issue though I still need to figure out why that happened.